Textures#
Textures in Ignis are patterns, nodes or other building blocks for shading networks.
All number and color parameters can be connected to a shading network or texture via PExpr to build an actual tree. Cycles are prohibited.
A texture is specified in the textures block with a name and a type. The type has to be one of the textures listed at this section below.
{
// ...
"textures": [
// ...
{"name":"NAME", "type":"TYPE", /* DEPENDS ON TYPE */},
// ...
]
// ...
}
Image texture (image)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
filename |
string |
None |
Path to a valid image file. |
|
filter_type |
string |
|
The filter type to be used. Has to be one of the following: [“bicubic”, “bilinear”, “nearest”]. |
|
wrap_mode |
string |
|
The wrap method to be used. Has to be one of the following: [“repeat”, “mirror”, “clamp”]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
|
linear |
boolean |
false |
The given image file is already in linear space and inverse gamma mapping can be skipped. Ignored for EXR and HDR images as it is expected that they are always in linear space. |
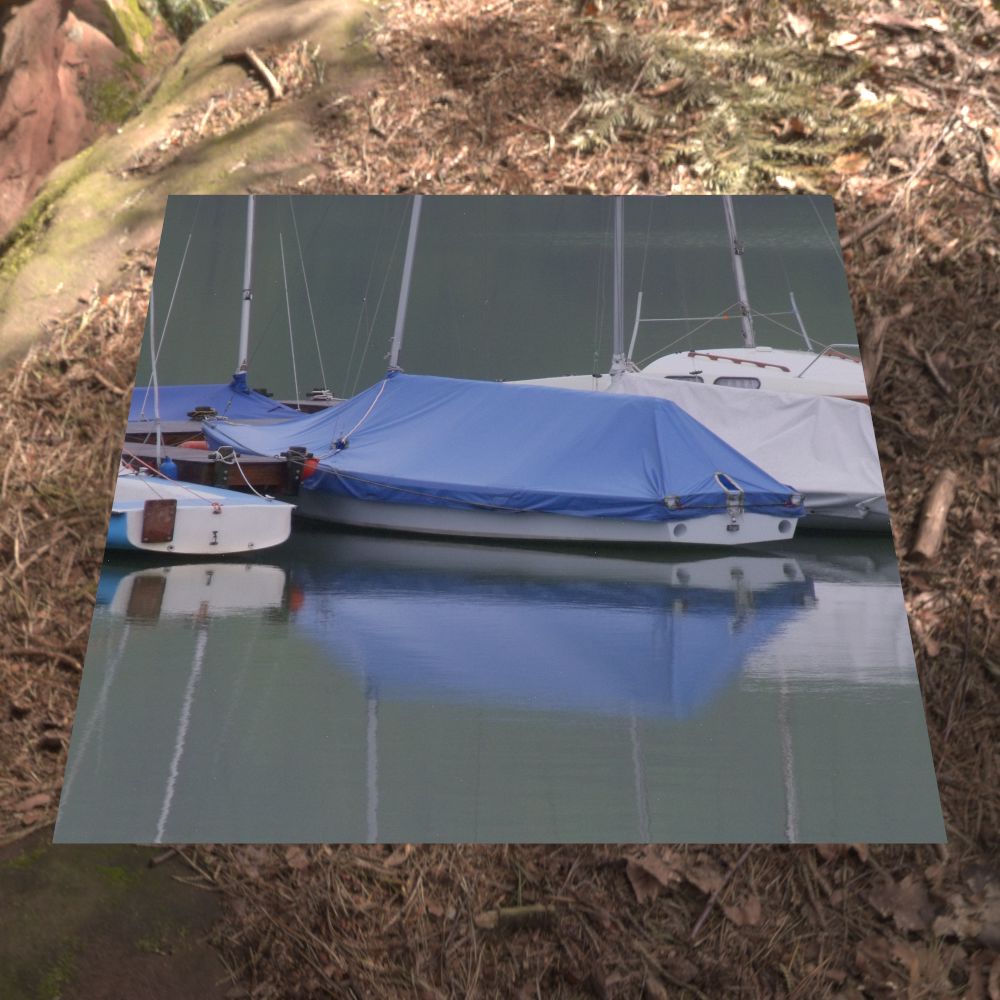
Image texture#
Brick (brick)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color0 color1 |
color |
|
The colors to used for the brick. color0 is the mortar, color1 is the actual brick. |
|
scale_x scale_y |
number |
|
Numbers of grids in a normalized frame [0,0]x[1,1]. |
|
gap_x gap_y |
number |
|
Normalized gap size. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
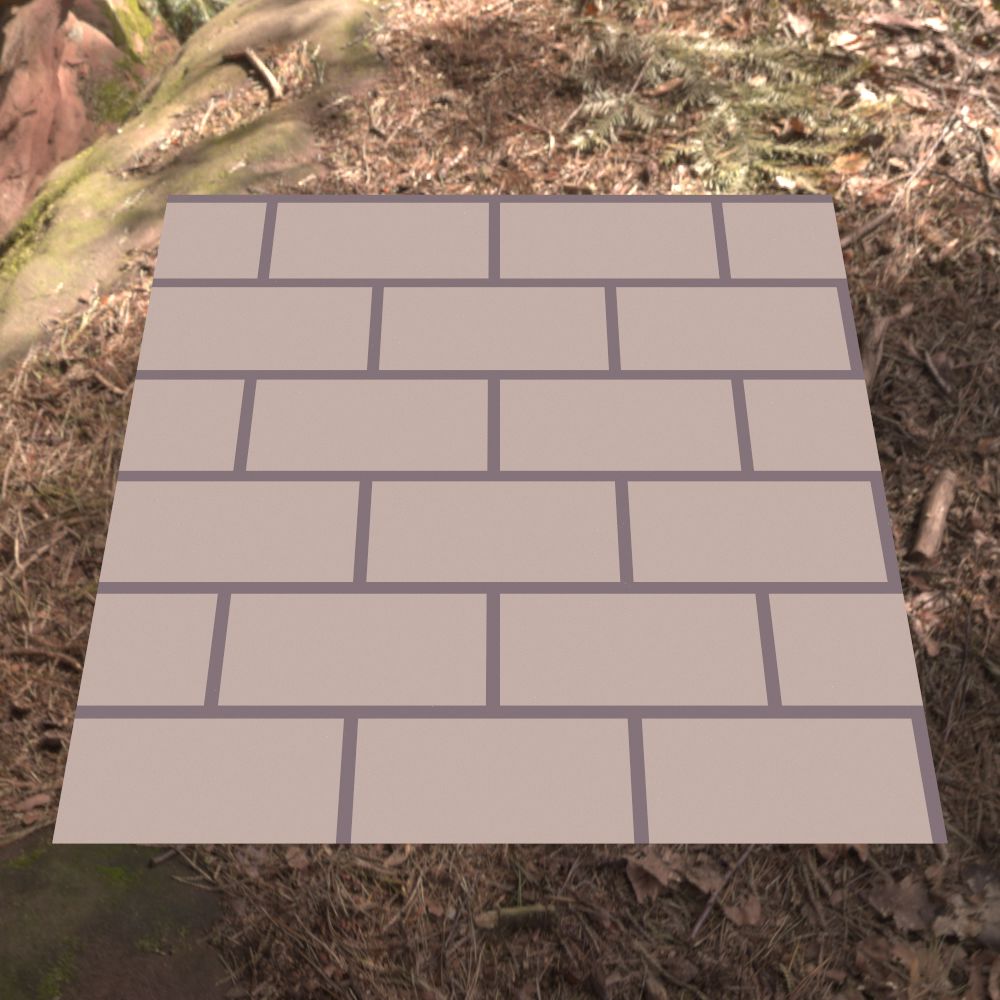
Brick texture#
Checkerboard (checkerboard)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color0 color1 |
color |
|
The colors to use in the checkerboard. |
|
scale_x scale_y |
number |
|
Numbers of grids in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
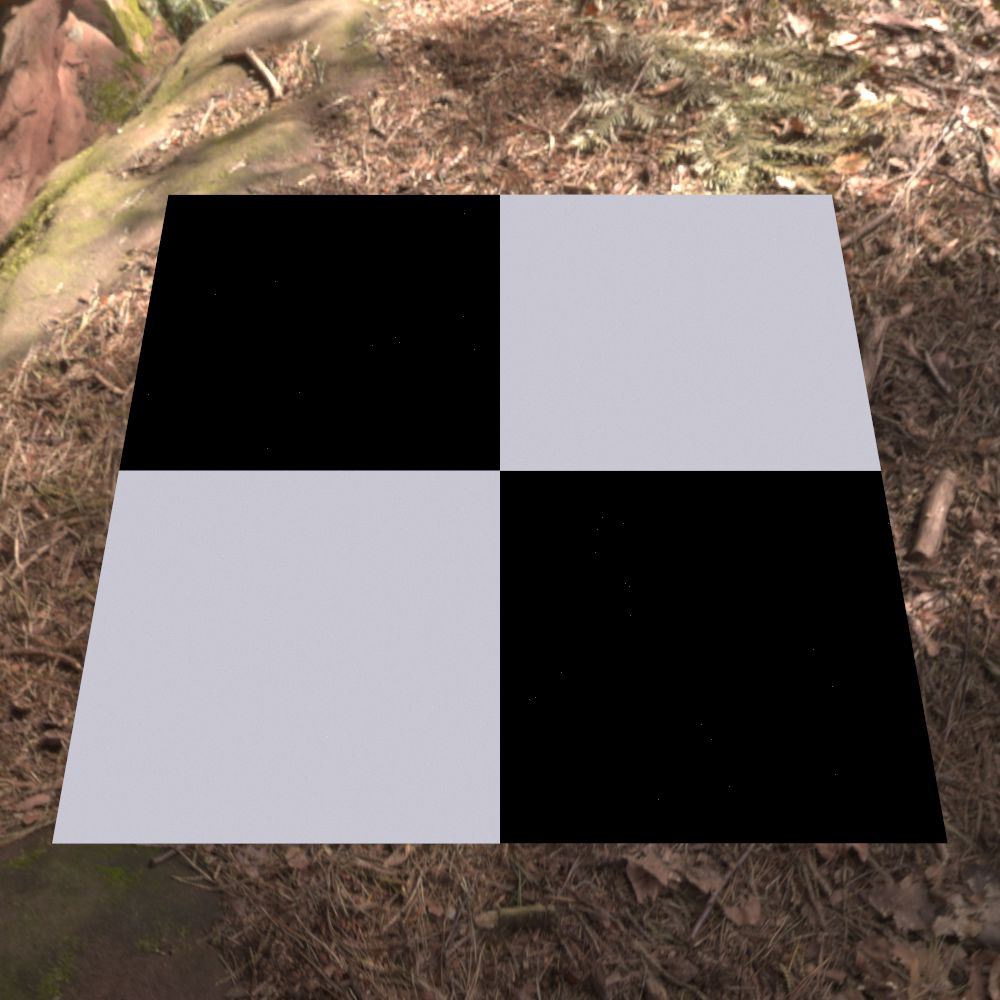
Checkerboard texture#
Noise (noise)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
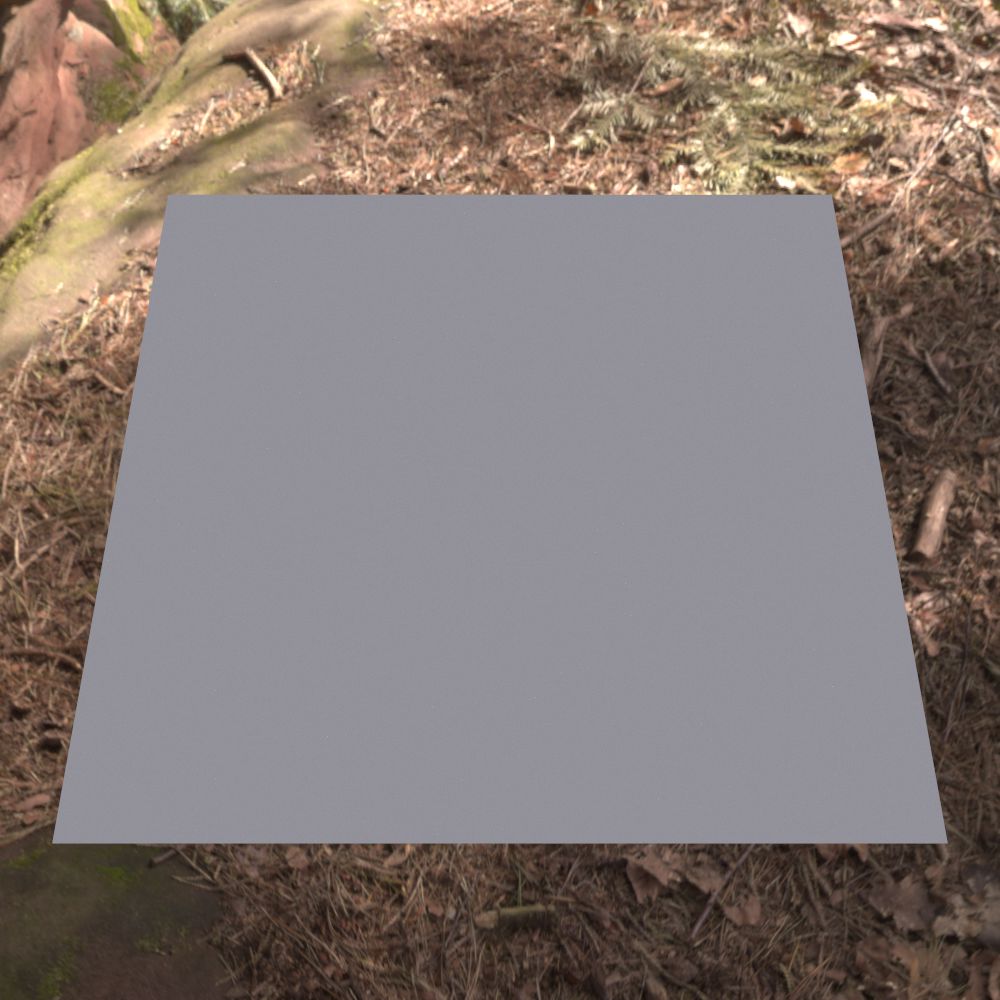
Noise texture, a slight color noise is visible#
Interpolated Noise (pnoise)#
Original noise used in legacy perlin implementation.
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
|
scale_x scale_y |
number |
|
Numbers of grids used for noise in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
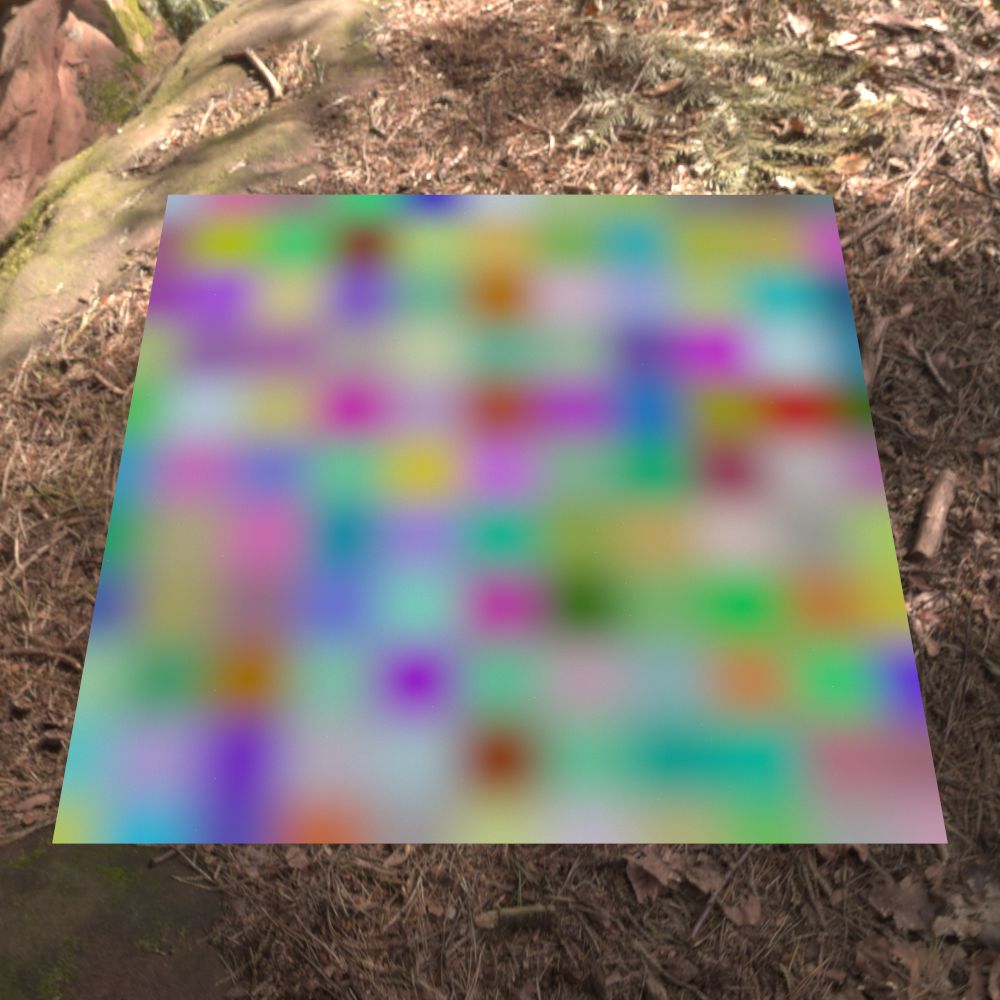
PNoise texture#
Cell Noise (cellnoise)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
|
scale_x scale_y |
number |
|
Numbers of grids used for noise in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
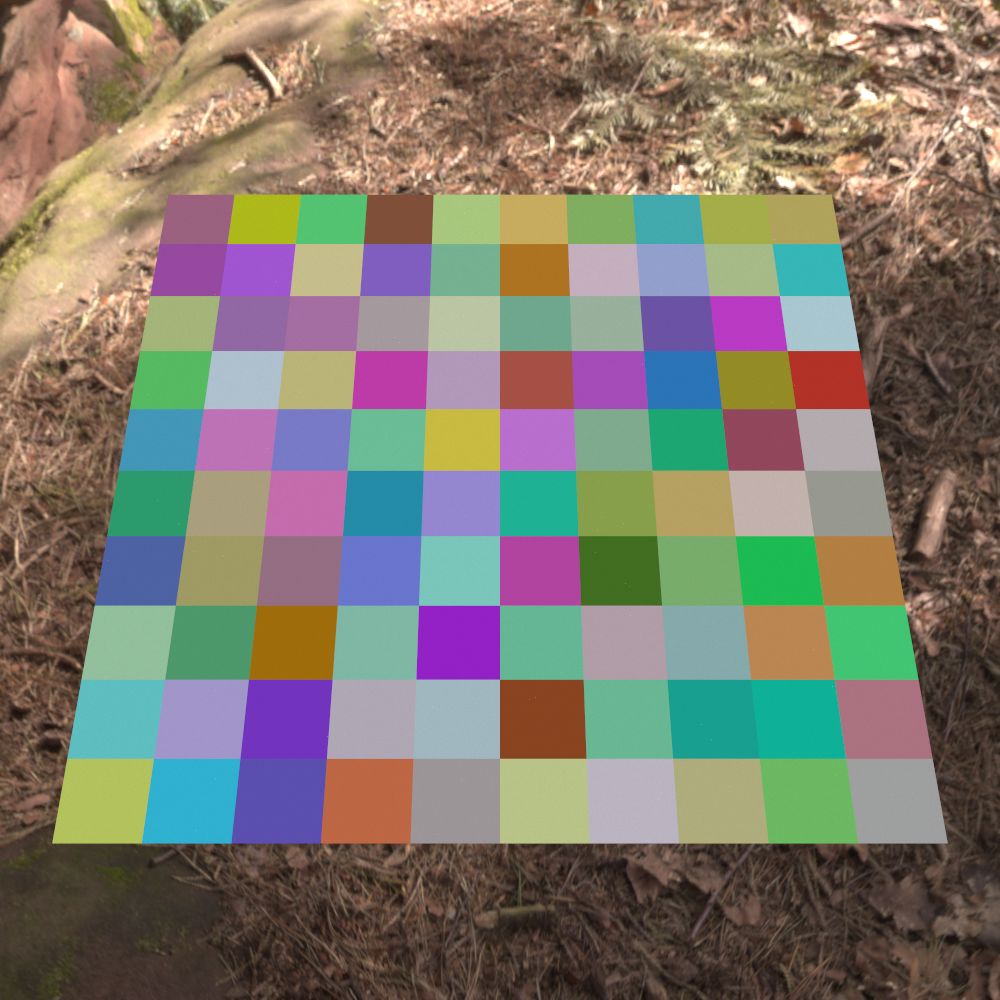
Cell noise texture#
Perlin Noise (perlin)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
|
scale_x scale_y |
number |
|
Numbers of grids used for noise in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
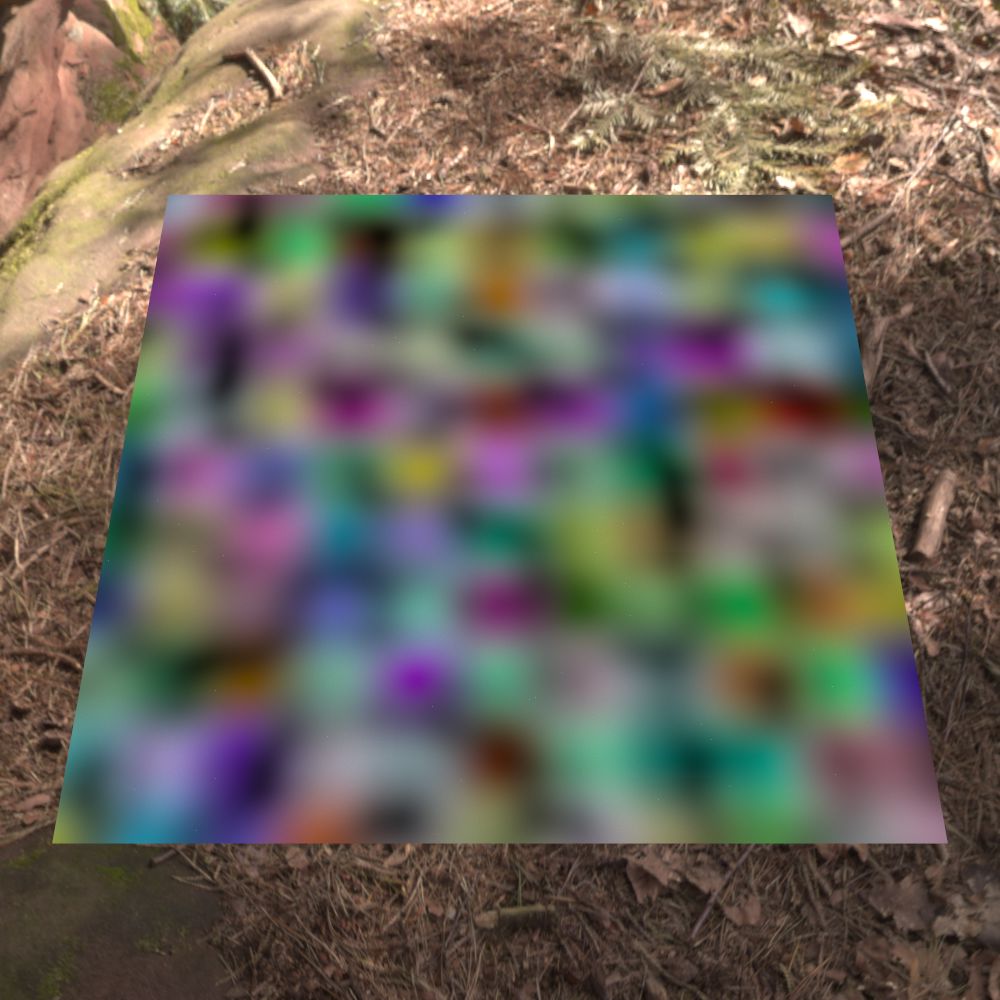
Perlin noise texture#
Voronoi Noise (voronoi)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
|
scale_x scale_y |
number |
|
Numbers of grids used for noise in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
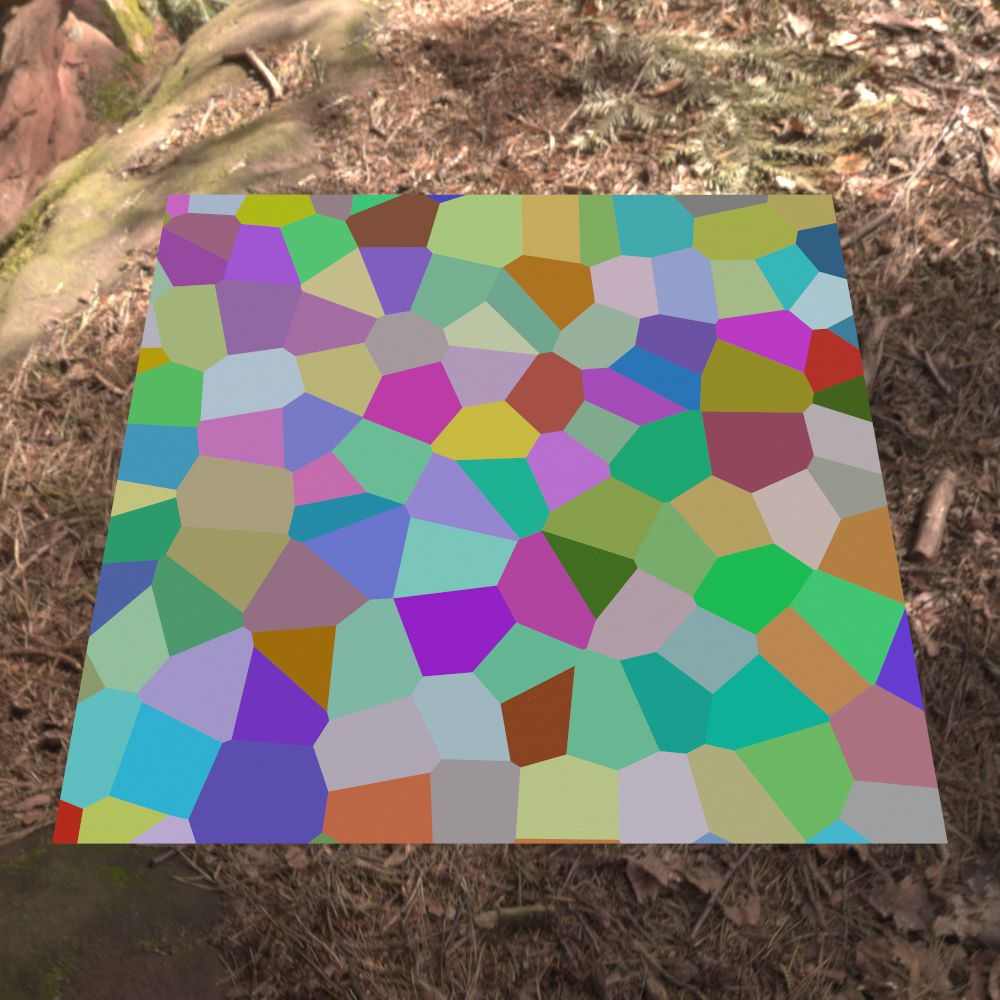
Voronoi texture#
Fractional Brownian Motion (fbm)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
color |
color |
|
Tint |
|
colored |
boolean |
false |
True will generate a colored texture, instead of a grayscale one. |
|
scale_x scale_y |
number |
|
Numbers of grids used for noise in a normalized frame [0,0]x[1,1]. |
|
transform |
transform |
Identity |
Optional 2d transformation applied to texture coordinates. |
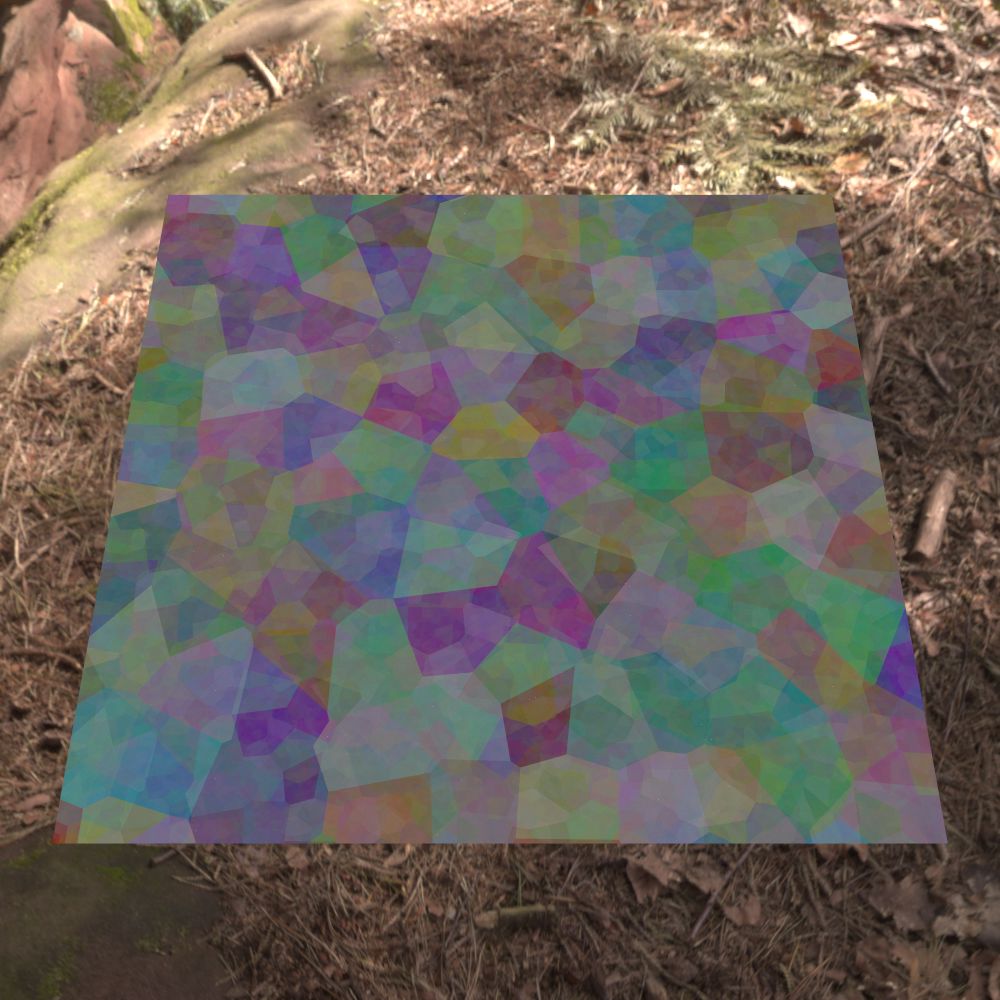
FBM texture#
Expression (expr)#
A custom PExpr expression with optional parameters.
Available are color (vec4
), vector (vec3
), number (num
) and bool
variables.
The parameters used inside the expression have to be prefixed with color_
, vec_
, num_
and bool_
respectively.
E.g., color_tint
will be called tint
inside the expression.
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
expr |
string |
None |
A PExpr based expression |
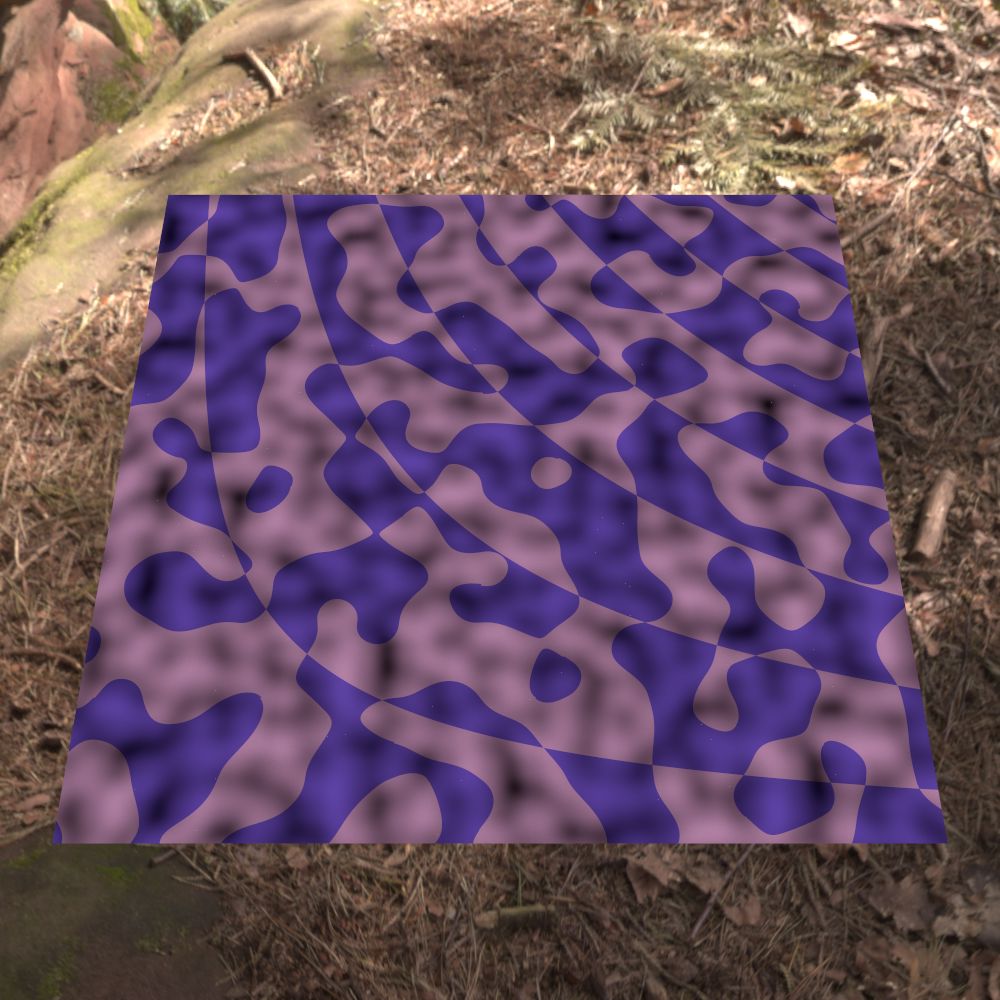
Custom texture generated by an expression#
{
"type": "expr",
"name": "tex",
"color_tint_1": [0.8, 0.4, 0.6],
"color_tint_2": [0.2, 0.1, 0.6],
"expr": "perlin(20*uv)*select(cos(8*Pi*uv.x*uv.y)*sperlin(10*uv) < 0, tint_1, tint_2)"
}
Texture transform (transform)#
Parameter |
Type |
Default |
PExpr |
Description |
---|---|---|---|---|
texture |
color |
None |
The texture the transform is applied to. |
|
transform |
transform |
Identity |
2d transformation applied to texture coordinates. |
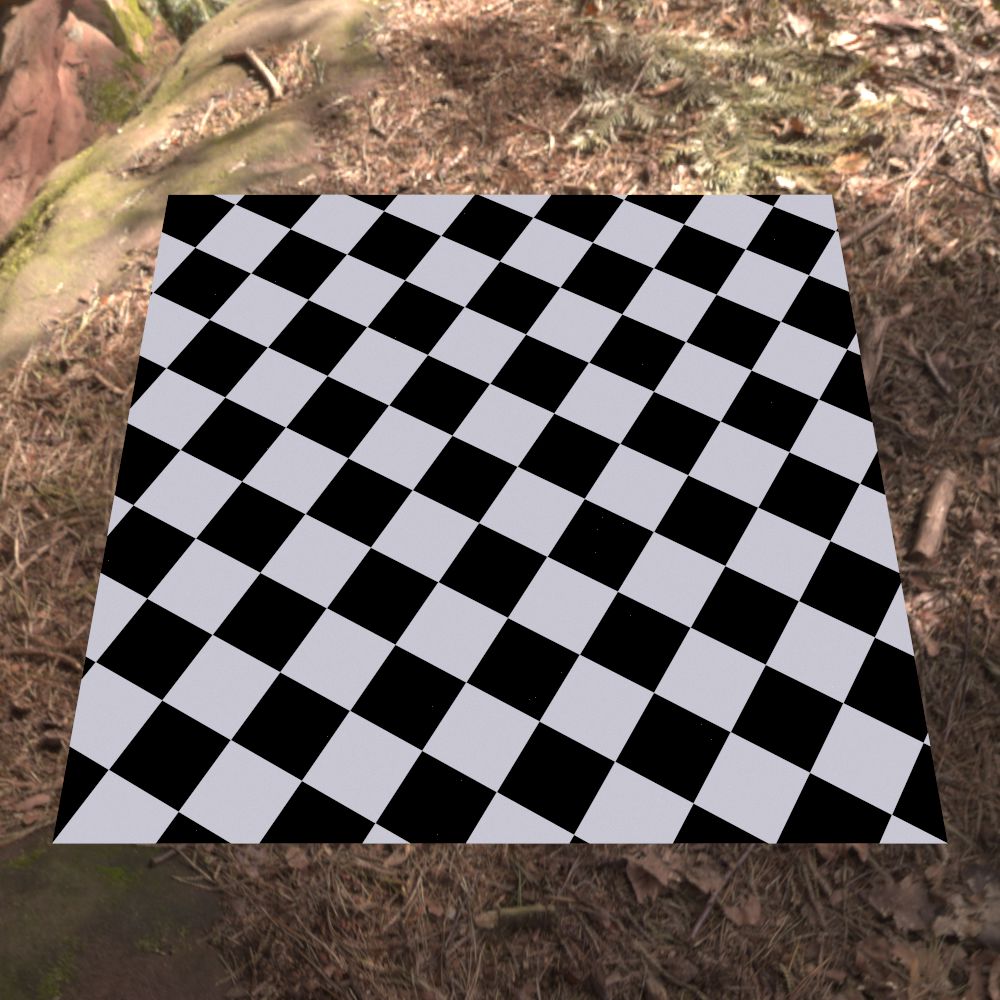
Transformed texture as a texture#