Shapes#
Shapes represent geometry in the scene.
A shape is specified in the shapes block with a name and a type. The type has to be one of the shape listed at this section below.
{
// ...
"shape": [
// ...
{"name":"NAME", "type":"TYPE", /* DEPENDS ON TYPE */},
// ...
]
// ...
}
Note
Shapes do not support PExpr expressions.
Triangle (triangle)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
p0 p1 p2 |
vector |
|
Vertices of the triangle. |
The parameters specified in Triangular Mesh can be used as well.
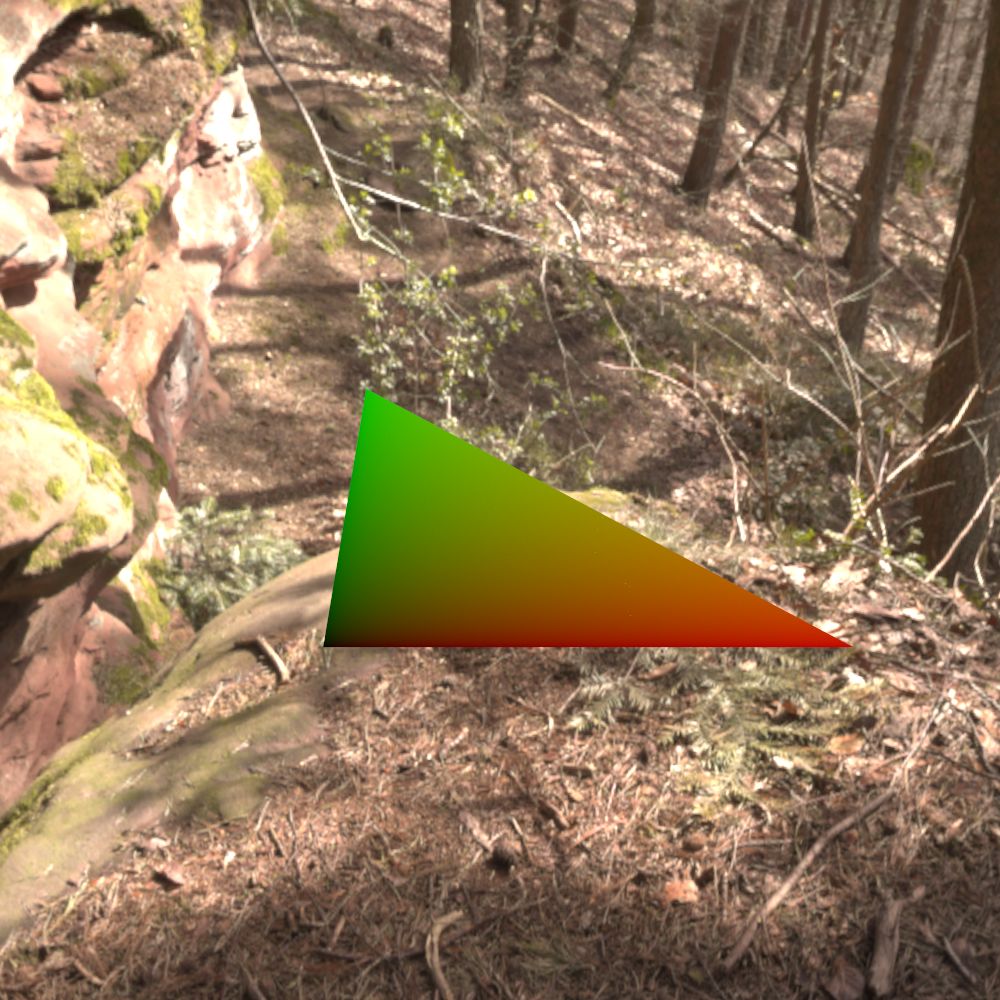
Triangle shape with visualized primitive coordinates#
Rectangle (rectangle)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
width height |
number |
|
Width and height of the rectangle in the XY-Plane. The rectangle is centered around the parameter origin. |
origin |
vector |
|
The origin of the rectangle. |
p0 p1 p2 p3 |
vector |
|
Vertices of the rectangle. This will only be used if no width or height is specified. |
The parameters specified in Triangular Mesh can be used as well.
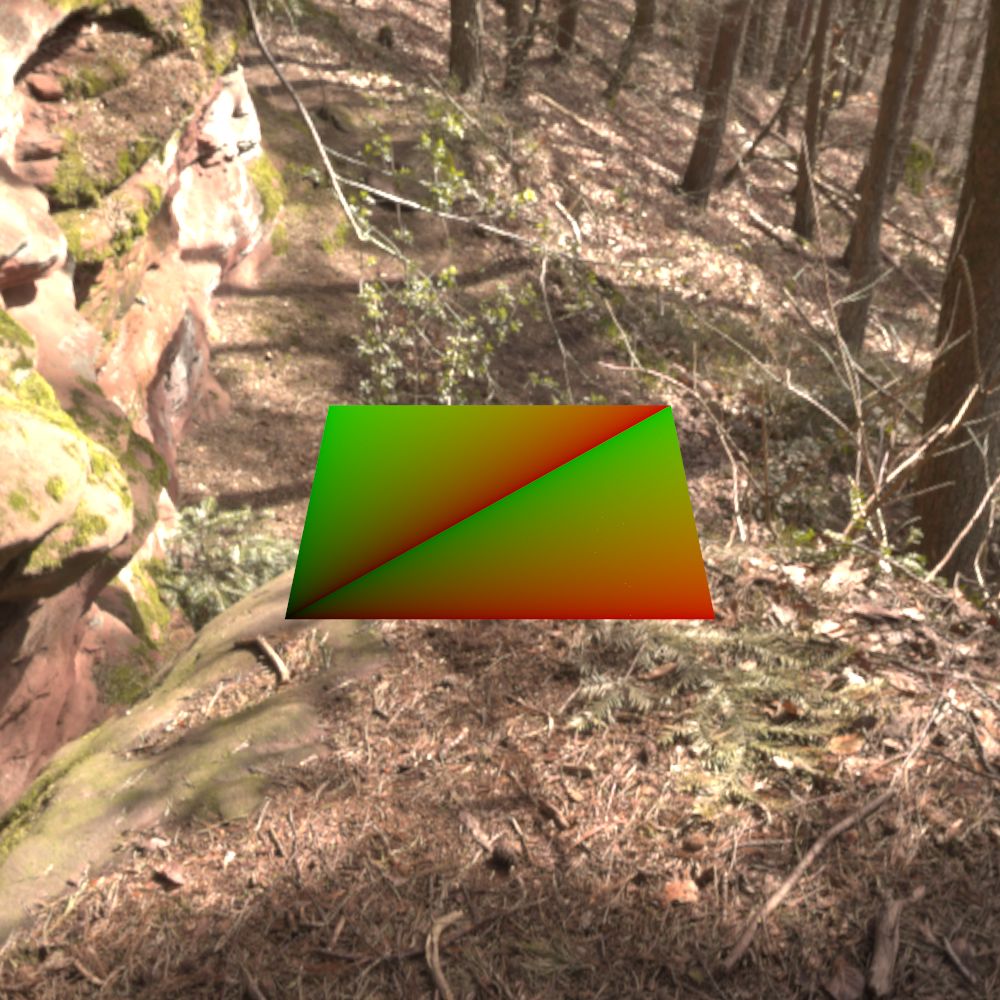
Rectangle shape with visualized primitive coordinates#
Box (box)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
width height depth |
number |
|
Width (x-axis), height (y-axis) and depth (z-axis) of the box. The box is centered around the parameter origin. |
origin |
vector |
|
The origin of the box. |
The parameters specified in Triangular Mesh can be used as well.
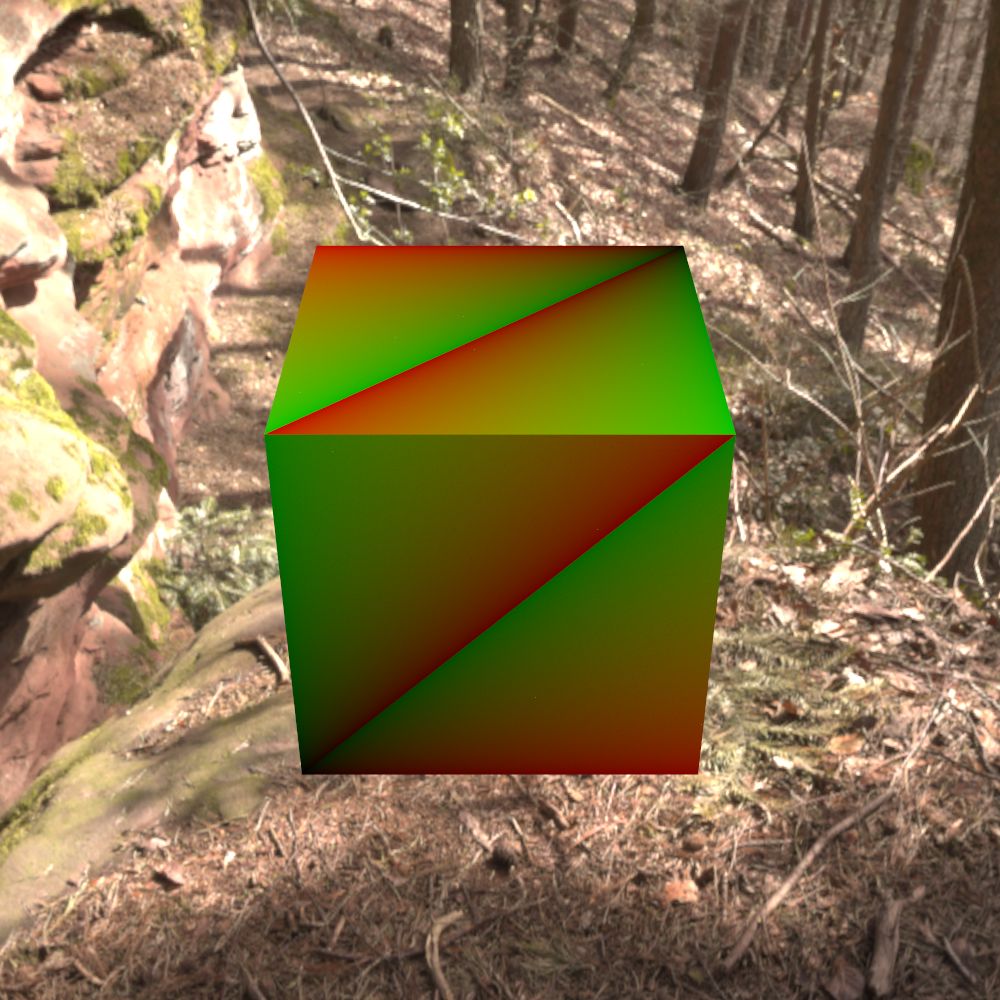
Box shape with visualized primitive coordinates#
Sphere (sphere)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the sphere. |
center |
vector |
|
The origin of the box. |
Note
In contrary to the other spheres, the constructed sphere is analytical and precise. Due to it not being constructed via triangle, the shape adds a new fundamental primitive type to the renderer, which in turn might decrease performance.
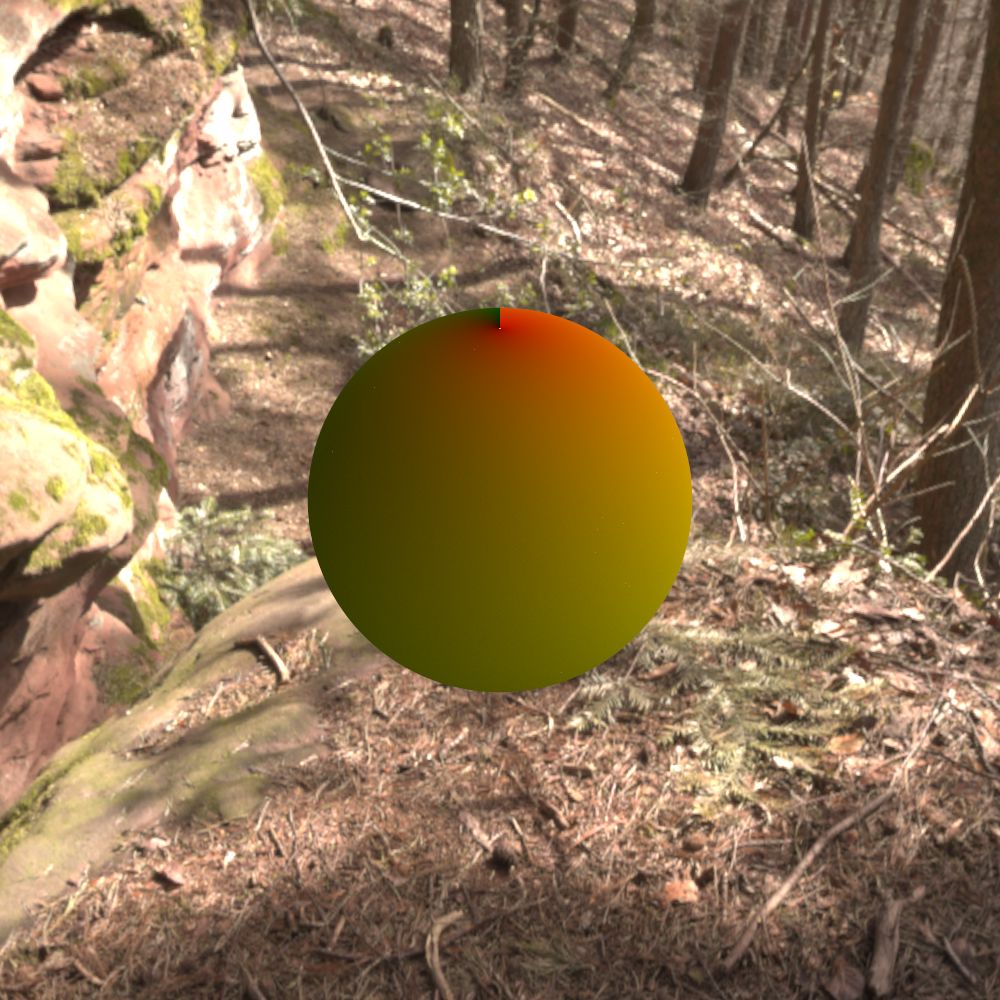
Sphere shape with visualized primitive coordinates. The primitive coordinates map to the normalized spherical coordinates#
Ico-Sphere (icosphere)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the sphere. |
center |
vector |
|
The origin of the box. |
subdivions |
integer |
|
Number of subdivions used. |
The parameters specified in Triangular Mesh can be used as well.
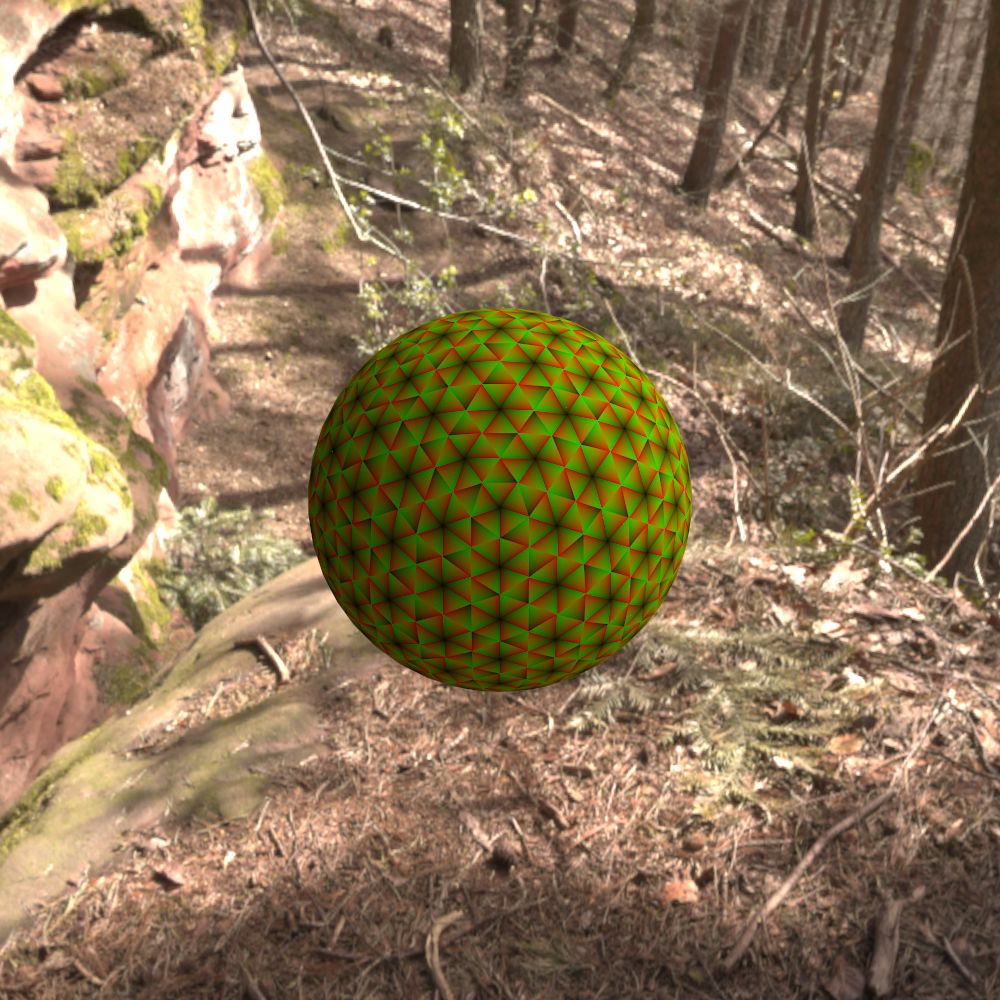
Ico sphere shape with visualized primitive coordinates. In contrary to the analytical sphere, the ico sphere consists of multiple tiny triangles#
UV-Sphere (uvsphere)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the sphere. |
center |
vector |
|
The origin of the box. |
stacks slices |
integer |
|
Stacks and slices used for internal triangulation. |
The parameters specified in Triangular Mesh can be used as well.
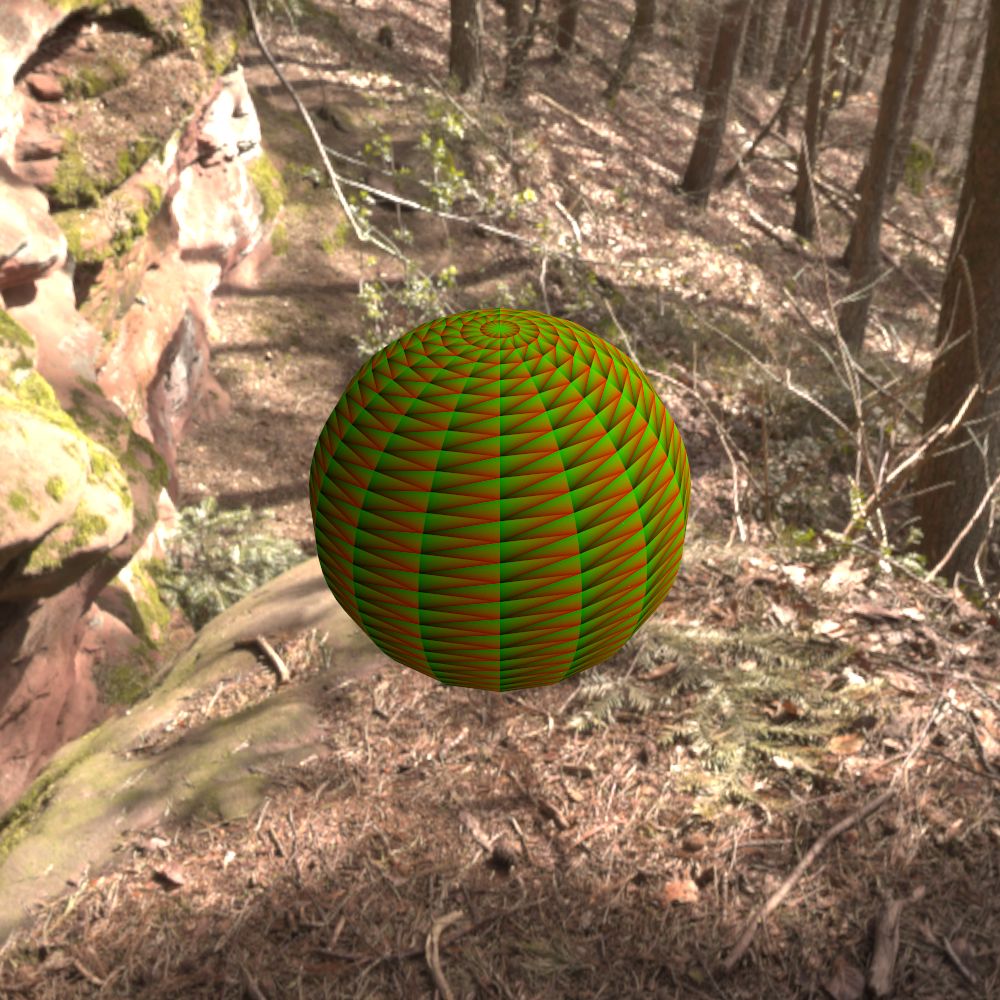
UV sphere shape with visualized primitive coordinates. In contrary to the analytical sphere, the uv sphere consists of multiple tiny triangles#
Cylinder (cylinder)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the cylinder for the top and bottom part. |
top_radius bottom_radius |
number |
|
Radius of the cylinder for the top and bottom part respectively. Can not be used together with radius. |
p0 p1 |
vector |
|
The origin of the top and bottom of the cylinder respectively. |
filled |
boolean |
true |
Set true to fill the top and bottom of the cylinder. |
sections |
integer |
|
Sections used for internal triangulation. |
The parameters specified in Triangular Mesh can be used as well.
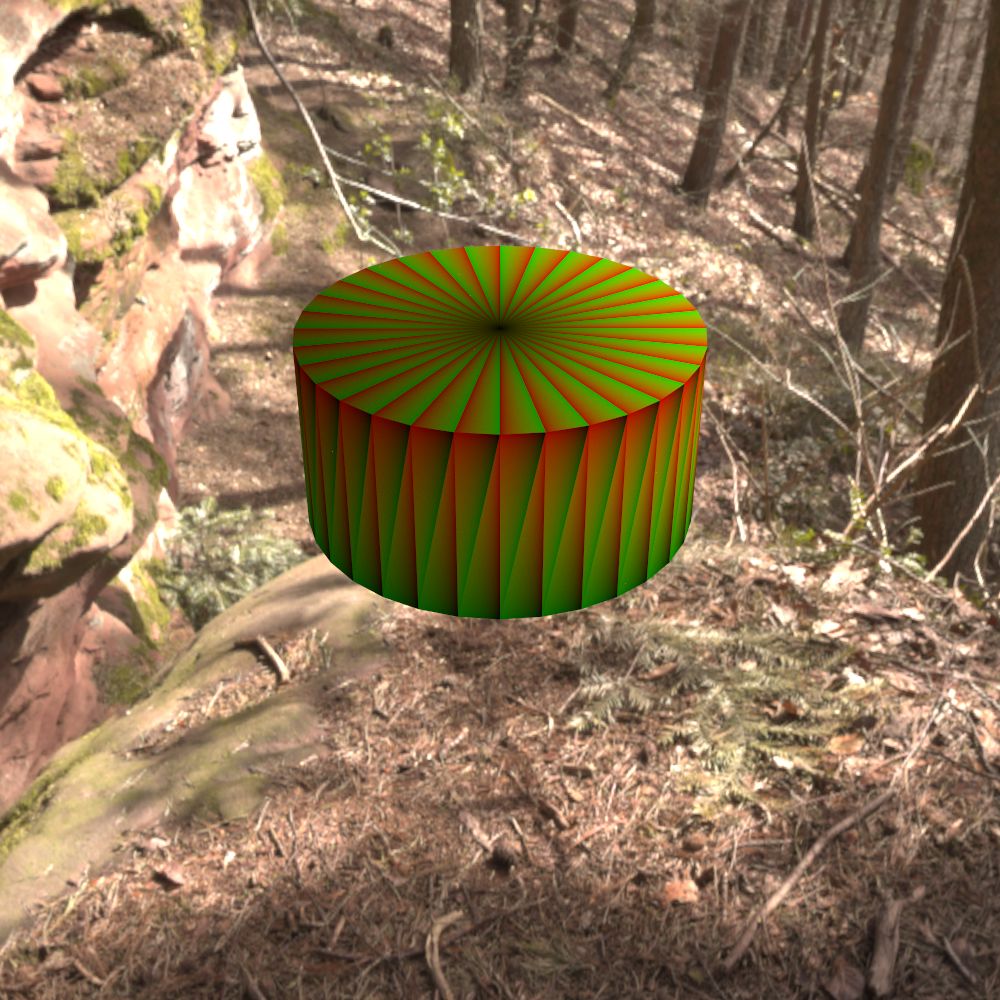
Cylinder shape with visualized primitive coordinates#
Cone (cone)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the cone. |
p0 p1 |
vector |
|
The origin of the top and bottom of the cone respectively. |
filled |
boolean |
true |
Set true to fill the bottom of the cone. |
sections |
integer |
|
Sections used for internal triangulation. |
The parameters specified in Triangular Mesh can be used as well.
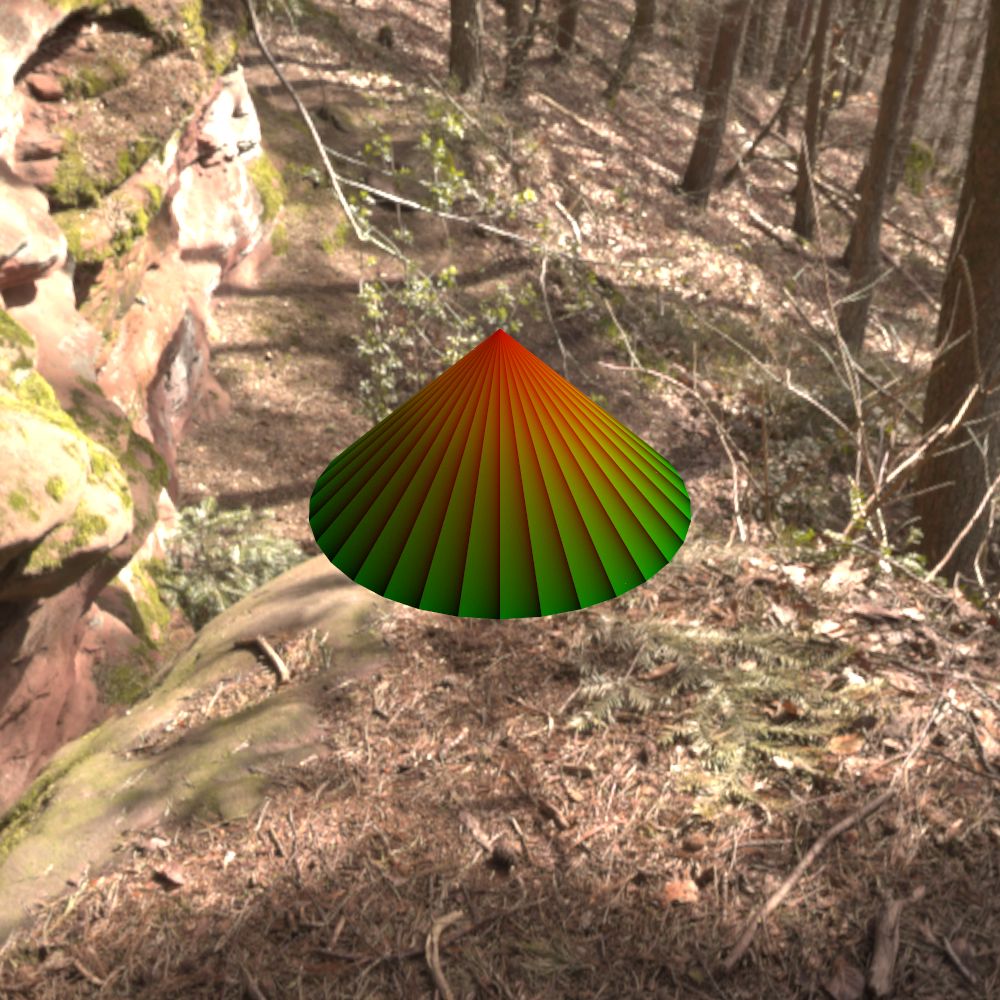
Cone shape with visualized primitive coordinates#
Disk (disk)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
radius |
number |
|
Radius of the disk. |
origin |
vector |
|
The origin of the disk. |
normal |
vector |
|
The normal of the disk. |
sections |
integer |
|
Sections used for internal triangulation. |
The parameters specified in Triangular Mesh can be used as well.
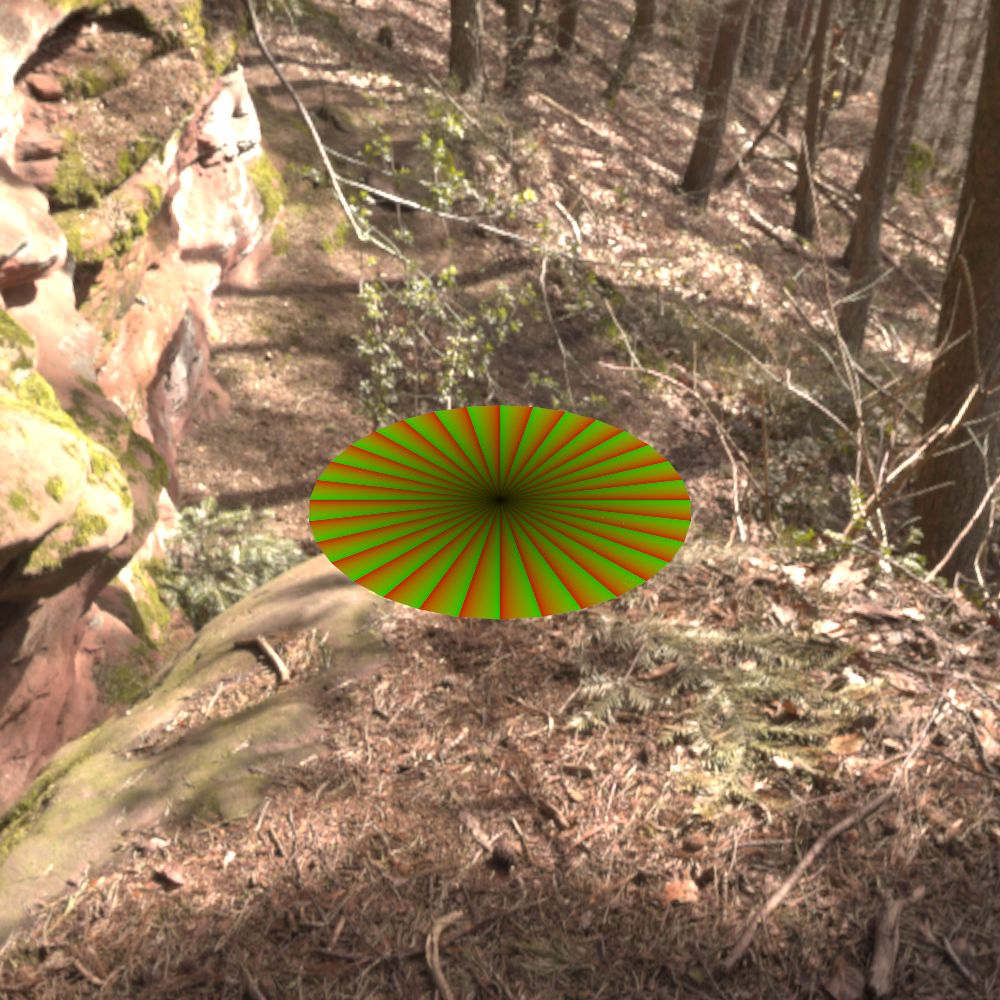
Disk shape with visualized primitive coordinates#
Wavefront Object Format (obj)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
filename |
string |
None |
Path to a valid .obj file. |
shape_index |
integer |
|
If greater or equal 0 a specific shape given by the index will be loaded, else all shapes will be merged to one. |
The parameters specified in Triangular Mesh can be used as well.
Polygon File Format (ply)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
filename |
string |
None |
Path to a valid .ply file. |
The parameters specified in Triangular Mesh can be used as well.
Mitsuba Serialized Format (mitsuba)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
filename |
string |
None |
Path to a valid .serialized or .mts file. |
shape_index |
integer |
|
A Mitsuba Serialized Format is able to contain multiple shapes. This parameter allows to select the requested one. |
The parameters specified in Triangular Mesh can be used as well.
External File (external)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
filename |
string |
None |
Path to a valid file with a known file extension. |
This type of shape will load a obj (.obj), ply (.ply) or mitsuba serialized mesh (.mts or .serialized) depending on the extension of the filename. Additional properties will be forwarded to the actual shape type.
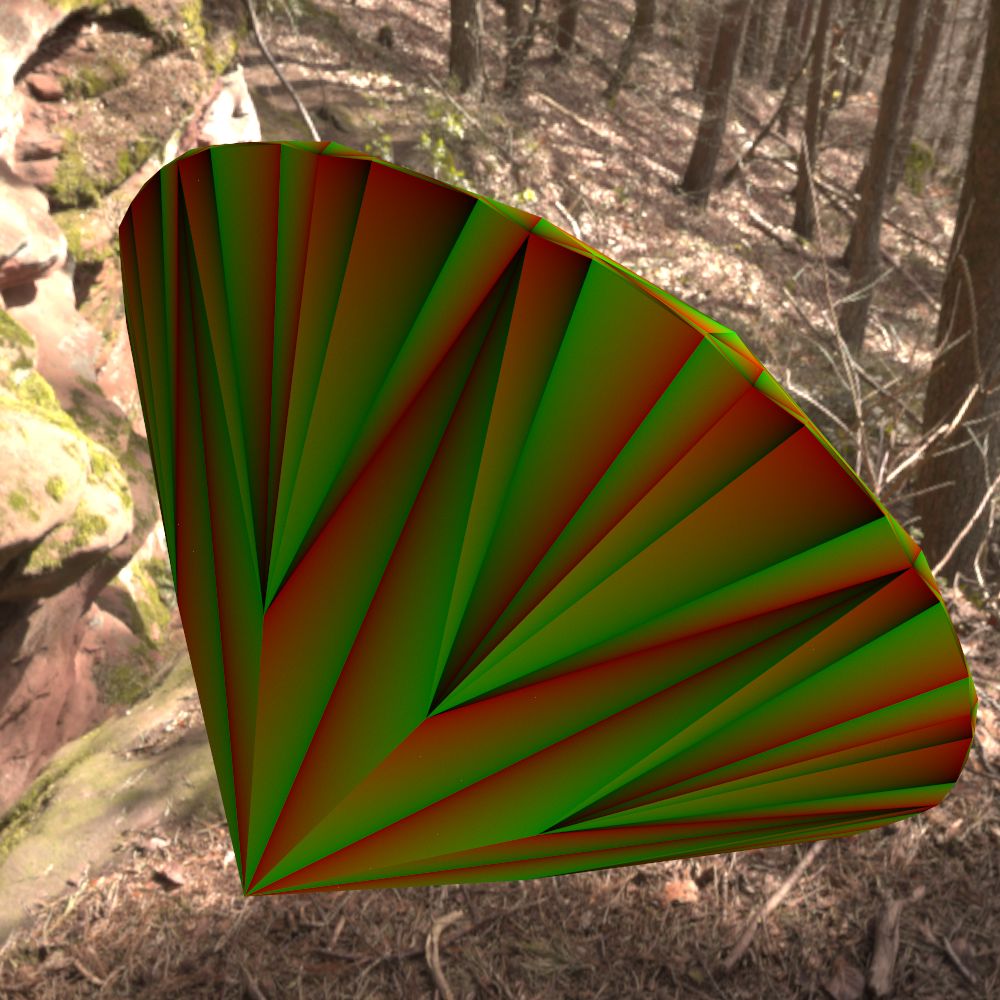
External shape (the diamond used in the showcase) with visualized primitive coordinates#
Inline Mesh (inline)#
Parameter |
Type |
Default |
Description |
---|---|---|---|
indices |
array of integer |
none |
Indices for a list of triangles given as a flat list of integers. The number of entries must be a multiple of 3. Only triangles are currently supported. |
vertices |
array of number |
none |
Three dimensional vertices given as a flat list of numbers. The number of entries must be a multiple of 3. |
normals |
array of number |
none |
Three dimensional normals given as a flat list of numbers. The number of entries must be a multiple of 3 and must match the number of vertices. |
texcoords |
array of number |
none |
Two dimensional texture coordinates given as a flat list of numbers. The number of entries must be a multiple of 2 and must match the number of vertices. |
The parameters specified in Triangular Mesh can be used as well.
Note
A list of number or integer can be specified via the {"type": "number", values=[...]}
with number
or integer
as type respectively.
Warning
It is recommended to use external files for large meshes, as the scene description is not intended to host large data. The inline should be used for interop between different frameworks and very small meshes.
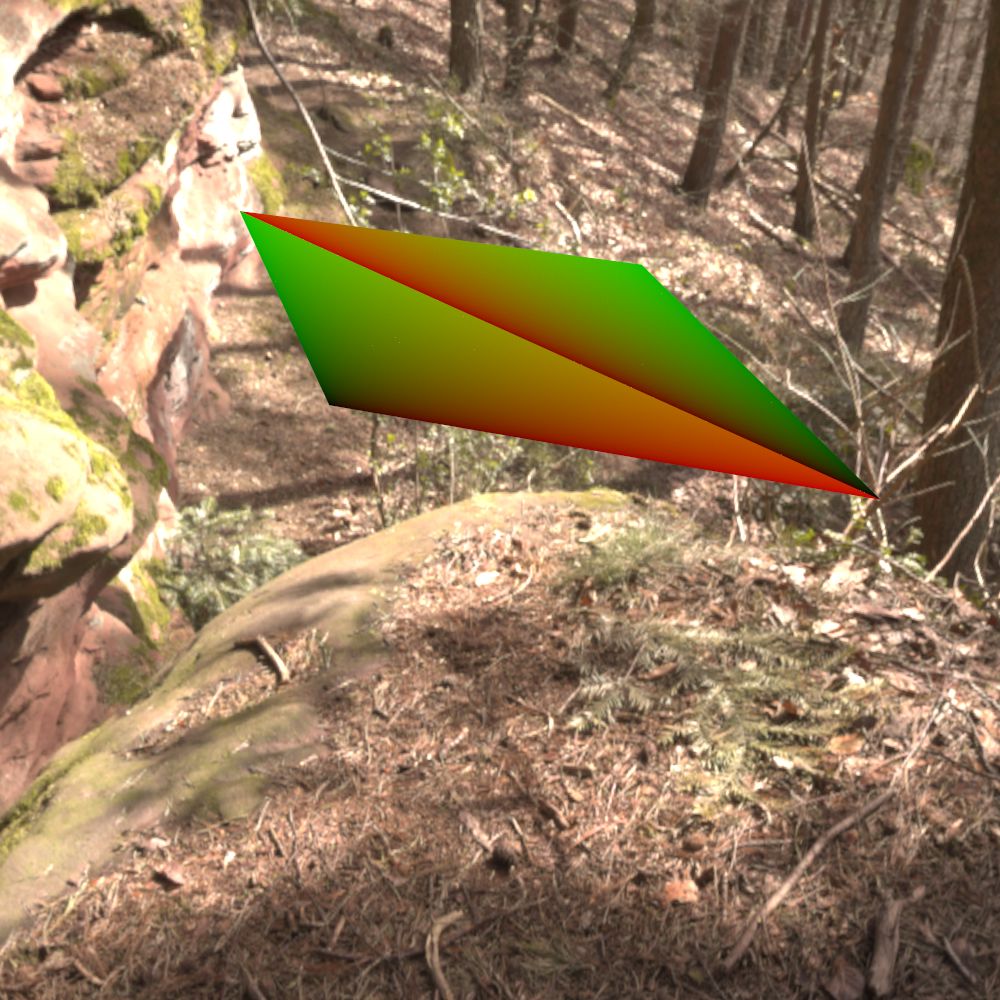
Inline custom plane specified directly in the scene file#
1{
2 "type": "inline",
3 "name": "Object",
4 "transform": [{"translate": [0,-2,2]}],
5 "indices": { "type": "integer", "values":[0,1,2, 1,2,3]},
6 "vertices": { "type": "number", "values":[-0.5,-0.5,0, 1,-1,0, -1,1,0, 0.5,0.5,0]}
7}
Triangular Mesh#
A triangular mesh specified above can have the following parameters additionally to the mentioned above as well:
Parameter |
Type |
Default |
Description |
---|---|---|---|
flip_normals |
boolean |
false |
Flip the normals. |
face_normals |
boolean |
false |
Use normals from triangles as vertex normals. This will let the object look hard. |
smooth_normals |
boolean |
false |
Use shared normals from triangles as vertex normals. This will let the object look smooth. |
generic_uv |
boolean |
false |
Compute normalized texture coordinates based on the local bounding box. Will replace previously defined texture coordinates. |
subdivision |
integer |
|
Number of triangular subdivisions to apply. |
refinement |
number |
|
Adaptive threshold. If greater than zero, triangles greater or equal than the threshold will be subdivided until all triangle areas are less than the given threshold. |
displacement |
string |
None |
Path to a image file containing grayscale values. This can not be an PExpr nor a reference to a texture. Use a plain filename. |
displacement_amount |
number |
|
Amount of displacement to apply. Can be negative to make the displacement inwards. |
transform |
transform |
Identity |
Apply given transformation to shape. |
Warning
Keep in mind that parameters like subdivision, refinement and displacement have a large impact on the performance of the loading process. If possible, the process should be precomputed with external software for large objects.
Note
Displacement within a shape is a tesselation step and creates a more dense mesh. Support for dynamic patterns and entities is planned for the future and will be implemented in the Entity interface.